Git quick reference
Why Git
Git is a fast, scalable, distributed revision control system with an unusually rich command set that provides both high-level operations and full access to internals.
help
git help
outline the most common use git commands.
$ git help
usage: git [--version] [--exec-path[=<path>]] [--html-path] [--man-path] [--info-path]
[-p|--paginate|--no-pager] [--no-replace-objects] [--bare]
[--git-dir=<path>] [--work-tree=<path>] [--namespace=<name>]
[-c name=value] [--help]
<command> [<args>]
The most commonly used git commands are:
add Add file contents to the index
bisect Find by binary search the change that introduced a bug
branch List, create, or delete branches
checkout Checkout a branch or paths to the working tree
clone Clone a repository into a new directory
commit Record changes to the repository
diff Show changes between commits, commit and working tree, etc
fetch Download objects and refs from another repository
grep Print lines matching a pattern
init Create an empty git repository or reinitialize an existing one
log Show commit logs
merge Join two or more development histories together
mv Move or rename a file, a directory, or a symlink
pull Fetch from and merge with another repository or a local branch
push Update remote refs along with associated objects
rebase Forward-port local commits to the updated upstream head
reset Reset current HEAD to the specified state
rm Remove files from the working tree and from the index
show Show various types of objects
status Show the working tree status
tag Create, list, delete or verify a tag object signed with GPG
See 'git help <command>' for more information on a specific command.
You can also use help
on a specific command, e.g. get help about commit
:
$ git help commit
Config commit user name and email
Set it globally:
$ git config --global user.name "Your Name"
$ git config --global user.email name@example.com
Or set per repo:
$ cd repo
$ git config user.name "Your Name"
$ git config user.email name@example.com
Initial a repo
$ mkdir /path/to/your/project
$ cd /path/to/your/project
$ git init
$ echo 'Hello' > README.md
$ git add .
$ git commit -m "First commit"
Commit changed files
Use status
to see modified/added/deleted files
$ git status
On branch feature/foo
Changes to be committed:
(use "git reset HEAD <file>..." to unstage)
deleted: lib/example.js
Changes not staged for commit:
(use "git add <file>..." to update what will be committed)
(use "git checkout -- <file>..." to discard changes in working directory)
modified: lib/index.js
Add changed files to commit by git add
:
$ git add lib/index.js
Check diff
to be committed (files after git add
):
$ git diff --cached
Check changes to be added by git diff
:
$ git diff
Commit changes to repo by git commit
:
$ git commit -m "comments here..."
Amend last commit message / author
Change the last commit message by git commit --amend
:
$ git commit --amend -m "new comments for last commit here..."
Change the last commit author by git commit --amend --author
:
$ git commit --amend --author "Foo <foo@example.com>"
Reset git history
You want to erase history after a specific commit.
You can erase history with git reset --hard <commit hash>
:
$ git reset --hard SHA1_HASH
Warning: reset --hard
is NOT revertible.
Checkout a specific commit
Jump to a old commit by checkout
:
$ git checkout SHA1_HASH
History
Check history by git log
:
$ git log
commit f82a22c19cbc32576f64f5d6b3f24b99ea8179c7
Author: Foo <foo@example.com>
Date: Tue May 16 17:11:22 2016 -0700
Fix typo
Check history with short hash, tag, branch graph:
$ git log --abbrev-commit --decorate --graph
Check history with diff by git log -p
:
$ git log -p
-p
output is verbose, it include diff for each changed file.
If you only want to see changed files summary, use:
$ git log --stat --summary
Check diff for a specific commit by git show
:
$ git show fb0902fa # `fb0902fa` here is a commit hash.
$ git show HEAD # the tip of the current branch
$ git show experimental # the tip of the "experimental" branch
Check diff in GUI by gitk
:
$ gitk
NOTE: gitk
required TK which is deprecated.
You can also use web interface to view history:
$ git instaweb
NOTE: instaweb
need a web server, for mac you can install lighttpd
which is recommended by git
:
$ brew install lighttpd
Branch
Create a new branch
$ git branch experimental
List branches
Use branch
to list local branches:
$ git branch
experimental
* master
*
stands for your current working branch.
List all branch:
$ git branch -a
List all remote branches:
$ git branch -r
Switch branch
Switch branch by checkout <branch name>
,
it can be local branch name or remote branch name:
$ git checkout experimental
Rename branch
Rename current branch name by -m
:
$ git branch -m <new branchname>
If new branchname is already exist, need use -M
to force rename instead of -m
.
Delete branch
If all the commits are merged, delete a branch by git branch -d
:
$ git branch -d experimental
If not all the commits are merged, force delete it by -D
:
$ git branch -d experimental
error: The branch 'experimental' is not fully merged.
If you are sure you want to delete it, run 'git branch -D experimental'.
$ git branch -D experimental
NOTE: -D
will not check merge, all the changes on branch will get lost.
Push local branch to remote repo
$ git checkout mybranch
$ git push origin mybranch
Checkout remote branch
$ git fetch
$ git branch -r # list remote branches
$ git checkout remote-branch1
Remove branch on remote repo
$ git push origin :mybranch # remove remote branch
$ git branch -d mybranch # also remove from local
orphan branch
orphan branch have no parents and it will be the root of a new history totally disconnected from all the other branches and commits.
Create a orphan branch:
git checkout --orphan <branchname>
This will create a new branch with no parents. Then, you can clear the working directory with:
git rm --cached -r .
Then add the documentation files, commit them and push them up to remote.
tag
Create tag
$ git tag 1.2.3 # without tag message
$ git tag -a 1.2.3 -m 'Release 1.2.3' # with tag message
Delete a tag
$ git tag –d <tagname>
NOTE: git tag -d
only delete local tag, it do not delete tag in remote.
To remove a remote tag, push a empty reference:
$ git push origin :tagname
Or use --delete
to remove remote tag:
$ git push --delete origin tagname
Example:
$ git push origin :2.1
To git@github.com:foo/bar.git
- [deleted] 2.1
List tags
$ git tag
v1.0
$ git tag -l 'v*' # filter tag
v4.3.1
Check tag detail
Check tag detail info, include commit hash and diff by git show
:
$ git show v1.0
Push tag to remote
By default, git push
does not push tags to remote, need use --tags
to push tags to remote:
$ git push --tags
Merge
--squash
- merge without branch history
Use --squash
to combine all the branch changes into a single commit,
it will totally erase branch commit history:
$ git checkout master
$ git merge --squash iss10
$ git commit -m "Merged iss10"
Rebase
In feature branch, use rebase instead of merge to avoid a merge commit in commit history:
$ git fetch
$ git rebase
$ git push -f
NOTE: rebase
will change commit history, so if you remote branch, you need push with -f
.
Remote
Add multiple remote
$ git remote add gitlab git@gitlab.com:foo/bar.git
$ git push gitlab master
Map local branch name to a different name on remote
git push
can change branch name on remote,
the following command will push local develop
branch to remote azure
as master
branch.
$ git push azure develop:master
.gitignore
Add files pattern to .gitignore
to indicate git not manage it.
Example of .gitignore
/node_modules
.DS_Store
.DS_Store?
._*
.Spotlight-V100
.Trashes
Icon?
ehthumbs.db
Thumbs.db
*.log
#
# xcode user settings
#
xcuserdata
.gitignore
is per project setting, use git config
to specific a global gitignore file:
$ git config --global core.excludesfile ~/.gitignore_global
Reference
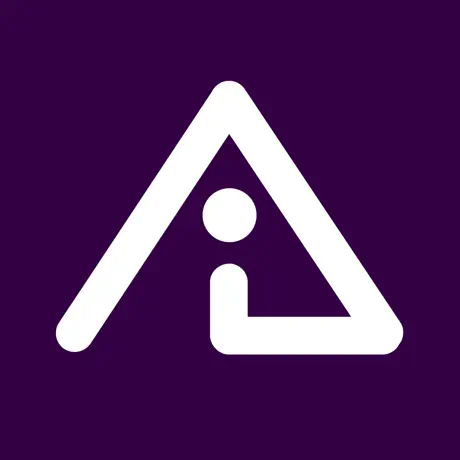
OmniLock - Block / Hide App on iOS
Block distractive apps from appearing on the Home Screen and App Library, enhance your focus and reduce screen time.
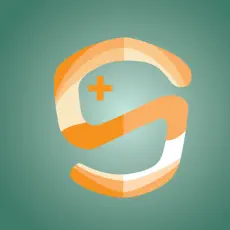
DNS Firewall for iOS and Mac OS
Encrypted your DNS to protect your privacy and firewall to block phishing, malicious domains, block ads in all browsers and apps