Swift Data init with heap buffer from C and free in Swift
Data
Take Ownership of a Raw Buffer Allocated by C on the Heap Without Recreating the BufferCan Swift Data
Take Ownership of a Raw Buffer Allocated by C on the Heap Without Recreating the Buffer?
Description
In Swift, you might encounter situations where you need to work with raw buffers allocated in C, especially when interfacing with C libraries or performing low-level memory management. A common question is whether Swift’s Data
type can take ownership of a raw buffer allocated by C on the heap without having to recreate or copy the buffer.
Swift’s Data type can take ownership of a raw buffer allocated by C on the heap without recreating the buffer. You can use the Data(bytesNoCopy:length:deallocator:)
initializer to achieve this. This initializer allows you to provide a pointer to the buffer and specify a deallocator to free the memory when the Data object is deallocated.
Use Case
Consider a scenario where you have a C function that allocates a buffer on the heap and returns a pointer to this buffer. You want to use this buffer in Swift without incurring the overhead of copying the data. By taking ownership of the buffer, you can manage its lifecycle directly in Swift, ensuring efficient memory usage and avoiding unnecessary data duplication.
Example
C Code
#include <stdlib.h>
#include <string.h>
void* allocateBuffer(size_t size) {
void* buffer = malloc(size);
if (buffer) {
memset(buffer, 0, size); // Initialize buffer to zero
}
// ...
return buffer;
}
Swift Code:
```swift
import Foundation
// Assume this function is provided by a C library
func allocateBuffer(size: Int) -> UnsafeMutableRawPointer?
// Function to create Data from a raw buffer
func dataFromCBuffer(size: Int) -> Data? {
guard let rawBuffer = allocateBuffer(size: size) else {
return nil
}
// Create Data from the raw buffer without copying
let data = Data(bytesNoCopy: rawBuffer, count: size, deallocator: .free)
return data
}
// Example usage
if let data = dataFromCBuffer(size: 1024) {
// Use the data
print("Data created with size: \(data.count)")
} else {
print("Failed to allocate buffer")
}
Explanation:
- C Function: The
allocateBuffer
function allocates a buffer on the heap and initializes it to zero. - Swift Function: The
dataFromCBuffer
function calls the C function to allocate the buffer and then creates a Data instance usingData(bytesNoCopy:count:deallocator:)
. - Ownership Transfer: By using the
.free
deallocator, Swift takes ownership of the buffer and ensures it is freed when theData
instance is deallocated.
This approach allows you to efficiently manage memory by avoiding unnecessary copies and ensuring that the buffer is properly deallocated when the Data object is no longer needed.
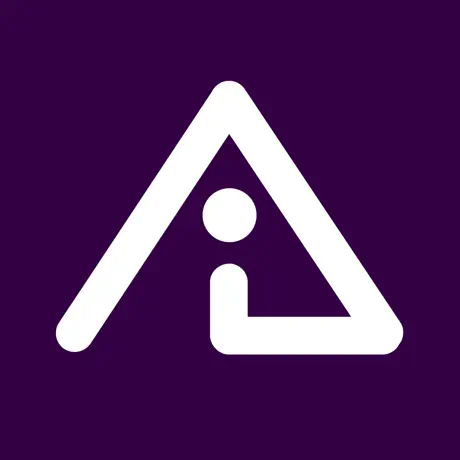
OmniLock - Block / Hide App on iOS
Block distractive apps from appearing on the Home Screen and App Library, enhance your focus and reduce screen time.
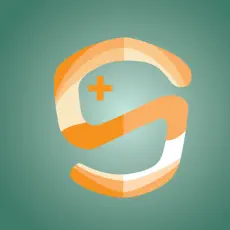
DNS Firewall for iOS and Mac OS
Encrypted your DNS to protect your privacy and firewall to block phishing, malicious domains, block ads in all browsers and apps