Get the IP address of a network interface by its name in Python on Linux an Mac OS X
How to get the IP address of a network interface by its name in Python on Linux and Mac OS X
Get the IP address of a network interface by its name in Python, on Linux
To get the IP address of a network interface by its name in Python, you can use the socket and fcntl modules. Here’s a step-by-step approach:
- Import the necessary modules.
- Create a socket.
- Use the fcntl.ioctl function to get the IP address of the specified network interface.
Here’s the code to achieve this:
# get_ip_linux.py
import socket
import fcntl
import struct
from typing import Optional
def get_ip_address(ifname: str) -> Optional[str]:
s = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
try:
ip_address = fcntl.ioctl(
s.fileno(),
0x8915, # SIOCGIFADDR
struct.pack('256s', ifname[:15].encode('utf-8'))
)[20:24]
return socket.inet_ntoa(ip_address)
except IOError:
return None
# Example usage
interface_name = 'eth0' # Replace with your network interface name
ip_address = get_ip_address(interface_name)
if ip_address:
print(f'The IP address of {interface_name} is {ip_address}')
else:
print(f'Could not get the IP address of {interface_name}')
Sample output:
The IP address of eth0 is 192.168.1.110
In this example:
- The get_ip_address function takes the network interface name (ifname) as an argument.
- A socket is created using socket.socket.
- The
fcntl.ioctl
function is used to get the IP address of the specified network interface. The0x8915
constant is the request code forSIOCGIFADDR
, which retrieves the address of the network interface. - The IP address is extracted from the result and converted to a human-readable format using socket.inet_ntoa.
- The function returns the IP address or None if an error occurs.
Replace ‘eth0’ with the name of your network interface to get its IP address.
Get the IP address of a network interface by its name in Python, on Mac OS X
import psutil
import socket
def get_ip_address(ifname: str) -> str:
addrs = psutil.net_if_addrs()
if ifname in addrs:
for addr in addrs[ifname]:
if addr.family == socket.AF_INET:
return addr.address
return None
# Example usage
ifname = 'en0' # Replace with your network interface name
ip_address = get_ip_address(ifname)
if ip_address:
print(f'The IP address of {ifname} is {ip_address}')
else:
print(f'Could not get the IP address of {ifname}')
Sample output:
The IP address of en0 is 192.168.1.119
Explanation:
- Import
psutil
: Import thepsutil
library. - Get Network Interface Addresses: Use
psutil.net_if_addrs()
to get a dictionary of network interfaces and their addresses. - Check Interface: Check if the specified interface name exists in the dictionary.
- Retrieve IP Address: Iterate through the addresses of the interface and return the IPv4 address.
- Handle Missing Interface: Return
None
if the interface or IPv4 address is not found.
Related pages:
- How to Parse URLs in Python: A Comprehensive Guide with Examples
- Python: How to print literal curly brace { or } in f-string and format string
- Python unicode string lowercase and caseless match
References
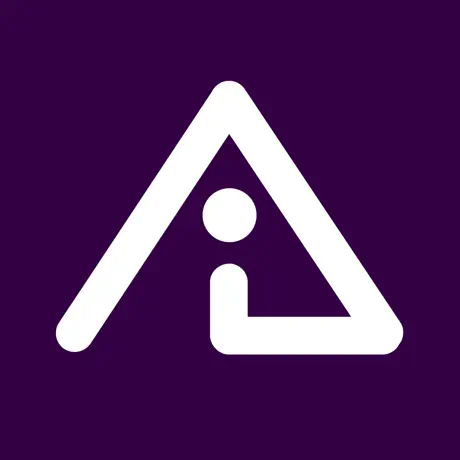
OmniLock - Block / Hide App on iOS
Block distractive apps from appearing on the Home Screen and App Library, enhance your focus and reduce screen time.
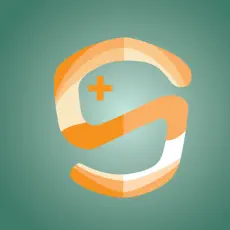
DNS Firewall for iOS and Mac OS
Encrypted your DNS to protect your privacy and firewall to block phishing, malicious domains, block ads in all browsers and apps
Ad