Python: How to print literal curly brace { or } in f-string and format string
A formatted string literal or f-string is a string literal that is prefixed with ‘f’ or ‘F’. These strings may contain replacement fields, which are expressions delimited by curly braces {}. While other string literals always have a constant value, formatted strings are really expressions evaluated at run time.
Formatted string literals or f-string was introduced in Python 3.6.
If you want to print literal curly brace without any escape, you will get following:
>>> print(f"{")
File "<stdin>", line 1
print(f"{")
^
SyntaxError: f-string: expecting '}'
>>> print(f"}")
File "<stdin>", line 1
print(f"}")
^
SyntaxError: f-string: single '}' is not allowed
\
can not be used escape, you will get SyntaxError
error:
>>> print(f"\{")
File "<stdin>", line 1
print(f"\{")
^
SyntaxError: f-string: expecting '}'
>>> print(f"\}")
File "<stdin>", line 1
print(f"\}")
^
SyntaxError: f-string: single '}' is not allowed
According f-string definition:
Escape sequences are decoded like in ordinary string literals (except when a literal is also marked as a raw string). After decoding, the grammar for the contents of the string is:
f_string ::= (literal_char | "{{" | "}}" | replacement_field)*
replacement_field ::= "{" f_expression ["="] ["!" conversion] [":" format_spec] "}"
f_expression ::= (conditional_expression | "*" or_expr)
("," conditional_expression | "," "*" or_expr)* [","]
| yield_expression
conversion ::= "s" | "r" | "a"
format_spec ::= (literal_char | NULL | replacement_field)*
literal_char ::= <any code point except "{", "}" or NULL>
The parts of the string outside curly braces are treated literally, except that any doubled curly braces
'{{'
or'}}'
are replaced with the corresponding single curly brace. A single opening curly bracket'{'
marks a replacement field, which starts with a Python expression.
The following code works:
>>> print(f"{{")
{
>>> print(f"}}")
}
>>> print(f"{{}}")
{}
>>> print(f"{{foo}}")
{foo}
This trick also apply to format string:
Format strings contain “replacement fields” surrounded by curly braces {}. Anything that is not contained in braces is considered literal text, which is copied unchanged to the output. If you need to include a brace character in the literal text, it can be escaped by doubling: {{ and }}.
This following example is example how to print literal curly brace '{'
in format string:
>>> print("{ {}".format("Foo"))
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ValueError: unexpected '{' in field name
>>> print("{{ {}".format("Foo"))
{ Foo
>>> print("}} {}".format("Foo"))
} Foo
>>> print("{{{{}}}} {}".format("Foo"))
{{}} Foo
>>> print("{{{}}} {}".format("Foo"))
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
IndexError: Replacement index 1 out of range for positional args tuple
Related pages:
- Get the IP address of a network interface by its name in Python on Linux an Mac OS X
- How to Parse URLs in Python: A Comprehensive Guide with Examples
- Python unicode string lowercase and caseless match
References
- https://docs.python.org/3/reference/lexical_analysis.html#f-strings
- https://docs.python.org/3/library/string.html#formatstrings
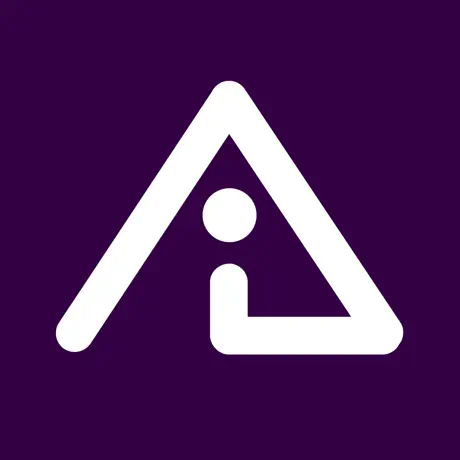
OmniLock - Block / Hide App on iOS
Block distractive apps from appearing on the Home Screen and App Library, enhance your focus and reduce screen time.
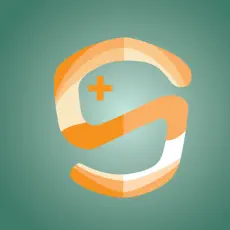
DNS Firewall for iOS and Mac OS
Encrypted your DNS to protect your privacy and firewall to block phishing, malicious domains, block ads in all browsers and apps