SwiftUI: How to Tap to Select All Text in a TextField on iOS
Problem
You have a TextField in your iOS app, and you want to automatically select all the text inside it when a user taps on it.
When working with iOS SwiftUI app development, you might encounter a common user interface requirement: the need to select or highlight all text within a `TextField`` when a user taps inside it. This behavior is similar to what you see in web browsers like Safari when you click inside the address bar. In this tutorial, we’ll explore how to achieve this functionality in your iOS app using Swift and SwiftUI.
Solution
We can accomplish this task by listening to the UITextField.textDidBeginEditingNotification
event and then using relevant UITextField
APIs to select all the text. There are two approaches to achieve this:
Approach 1: Using textField.selectAll()
In this approach, we’ll call the selectAll() method on the UITextField to select all the text when the text field begins editing. Here’s how you can implement it:
import SwiftUI
import Combine
TextField("Search key words...", text: $keywords)
.padding()
.onReceive(NotificationCenter.default.publisher(for: UITextField.textDidBeginEditingNotification)) { obj in
// Click to select all the text.
if let textField = obj.object as? UITextField {
textField.selectAll(nil)
}
}
With this code, when the user taps inside the text field, all the text will be automatically selected.
Approach 2: Using textField.selectedTextRange
In this approach, we’ll set the selectedTextRange property of the UITextField to select all the text. Here’s how you can implement it:
import SwiftUI
import Combine
TextField("Search key words...", text: $keywords)
.padding()
.onReceive(NotificationCenter.default.publisher(for: UITextField.textDidBeginEditingNotification)) { obj in
// Click to select all the text.
if let textField = obj.object as? UITextField {
textField.selectedTextRange = textField.textRange(from: textField.beginningOfDocument, to: textField.endOfDocument)
}
}
In this code, it will have the same effect as the first approach, selecting all the text when the text field begins editing.
Conclusion
Selecting all text in a TextField`` when a user taps inside it is a common user interface requirement in iOS app development. By using the
UITextField.textDidBeginEditingNotification` event and appropriate UITextField APIs, you can easily achieve this functionality and provide a seamless user experience in your iOS app.
Related pages:
- Swift Data init with heap buffer from C and free in Swift
- DispatchQueue QoS Order Explained Best Practices & Common Pitfalls to Avoid
- iOS RUNNINGBOARD 0xdead10cc crash troubleshooting
- iOS objc_retain crash troubleshooting
- Direct Download Link for All Xcode Releases/Beta, Compilers and SDK Information
- Guide: Jailbreaking Apple TV 4K
- iOS Security: App security in iOS and iPadOS - code signing, entitlement and sandbox etc.
- Tips on Use Swift Package Manager (SPM) with Continuous Integration / Delivery (CI/CD)
- Jailbreak iPhone 8 iOS 16.2 with palera1n and use frida dump to decrypt ipa
- Use frida and objection to penetration test iOS app security
- Troubleshooting: iOS auto layout warning about UIView-Encapsulated-Layout-Height
- Troubleshooting: loading carthage framework error: dyld: Library not loaded: @rpath/...
- What's new in SwiftUI iOS 16.4 beta 2
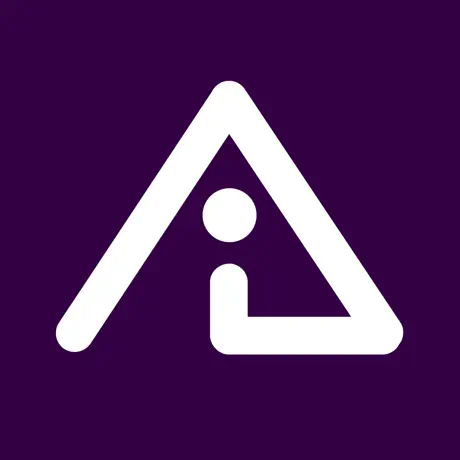
OmniLock - Block / Hide App on iOS
Block distractive apps from appearing on the Home Screen and App Library, enhance your focus and reduce screen time.
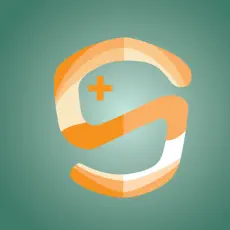
DNS Firewall for iOS and Mac OS
Encrypted your DNS to protect your privacy and firewall to block phishing, malicious domains, block ads in all browsers and apps